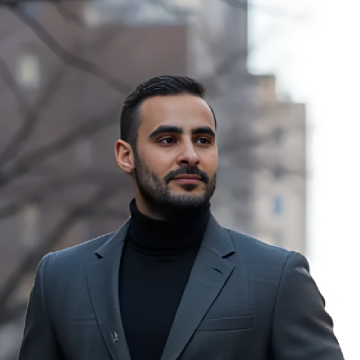
Amr Tarek
Software Engineer
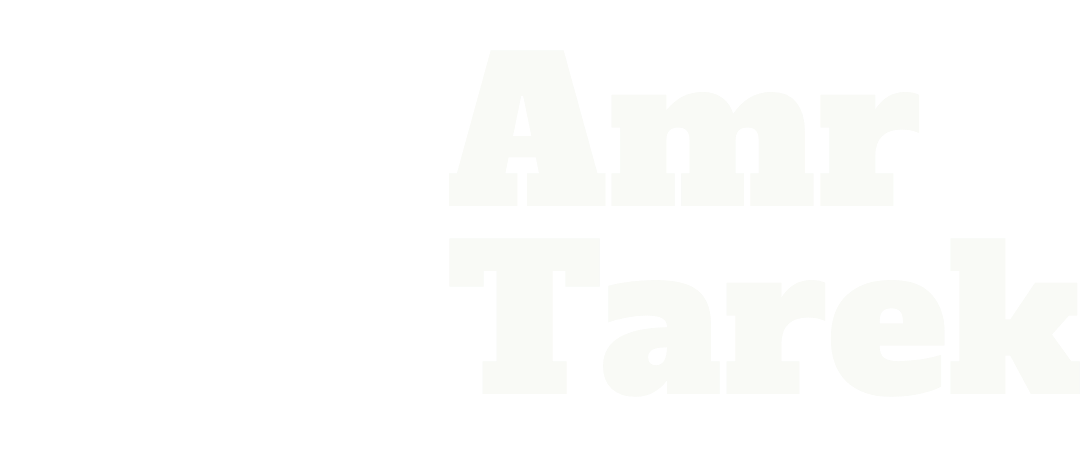
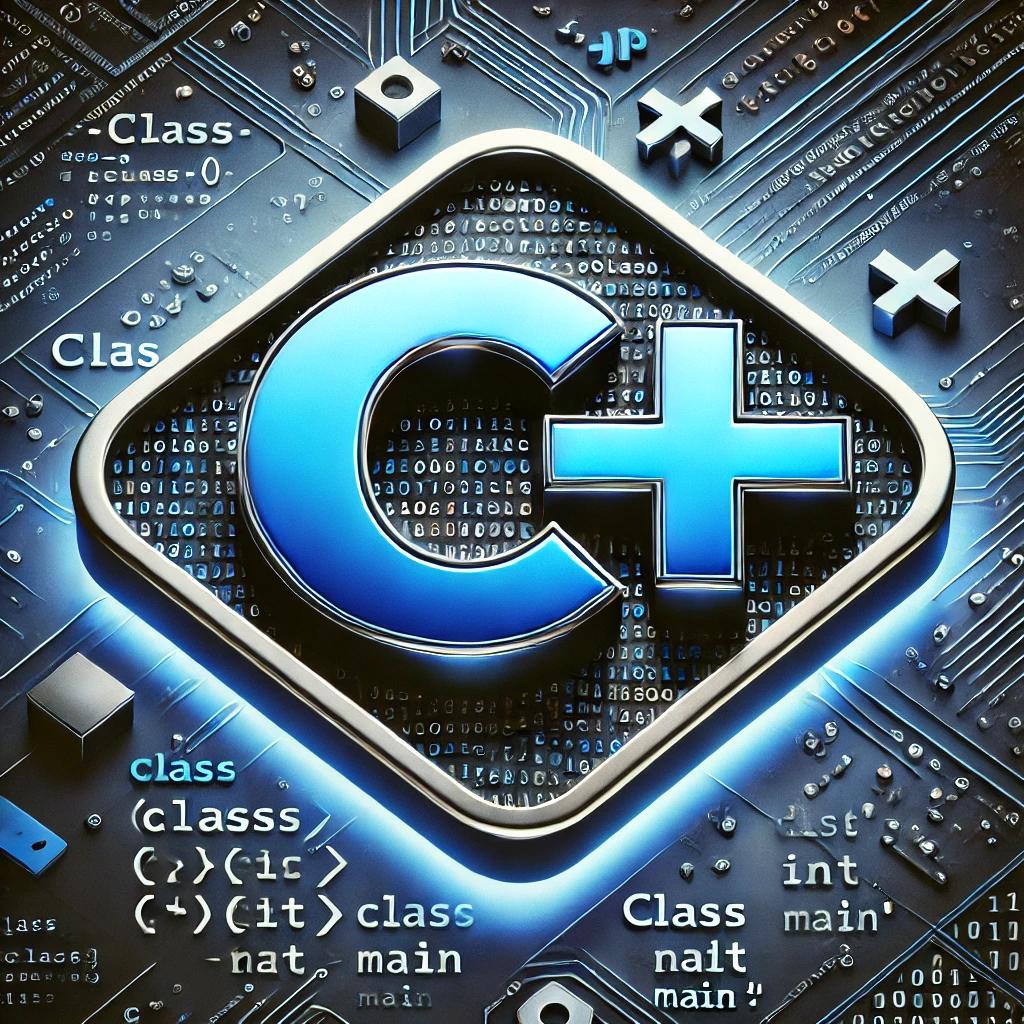
C++ STL - Iterators
iterators are objects that point to elements within a container (like arrays, vectors, or lists) and allow you to traverse the container's elements in a standardized way.
Iterators are central to the C++ Standard Template Library (STL) and provide a uniform interface for accessing container elements, whether the container is sequential (like a vector) or associative (like a map or set).
They are an objects like a pointer that points to an element inside a container
Use iterator to deal with container rather than pointers because it is safer and it will prevent the known behaviour in case of the container is empty.
Types of Iterators
Iterators come in different categories depending on how you can move through the container:
- Input Iterator: Can read from the pointed element.
- Output Iterator: Can write to the pointed element.
- Forward Iterator: Can move forward (increment) through the container.
- Bidirectional Iterator: Can move both forward (increment) and backward (decrement).
- Random Access Iterator: Can jump directly to any element (e.g.,
std::vector
iterator).
Basic Example of Using Iterators
Let’s say you have a std::vector<int>
and want to iterate over its elements using iterators:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
// Define an iterator for the vector
std::vector<int>::iterator it;
// Traverse the vector
for (it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " "; // Dereference the iterator to access the value
}
return 0;
}
Creating Your own iterator
Creating a custom iterator requires defining a class that mimics the behavior of STL iterators.
The class should implement certain functions like operator*()
for dereferencing and operator++()
for advancing the iterator. Here’s a basic example:
- *Dereferencing (`operator`)**: This returns the current element the iterator points to.
- Increment (
operator++
): Moves the iterator to the next element in the container. - Comparison operators (
operator==
,operator!=
): Allow comparing iterators to know when to stop iterating (e.g.,it != end
).
#include <iostream>
template<typename T>
class MyIterator {
private:
T* ptr; // Pointer to the current element
public:
// Constructor
MyIterator(T* p = nullptr) : ptr(p) {}
// Dereference operator
T& operator*() const {
return *ptr;
}
// Prefix increment operator (++it)
MyIterator& operator++() {
++ptr;
return *this;
}
// Postfix increment operator (it++)
MyIterator operator++(int) {
MyIterator temp = *this;
++ptr;
return temp;
}
// Equality operator (it == other)
bool operator==(const MyIterator& other) const {
return ptr == other.ptr;
}
// Inequality operator (it != other)
bool operator!=(const MyIterator& other) const {
return ptr != other.ptr;
}
};
int main() {
int numbers[] = {1, 2, 3, 4, 5};
// Create iterators pointing to the start and end of the array
MyIterator<int> begin(numbers);
MyIterator<int> end(numbers + 5);
// Traverse the array using the custom iterator
for (MyIterator<int> it = begin; it != end; ++it) {
std::cout << *it << " ";
}
return 0;
}