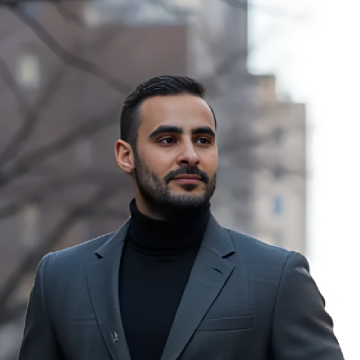
Amr Tarek
Software Engineer
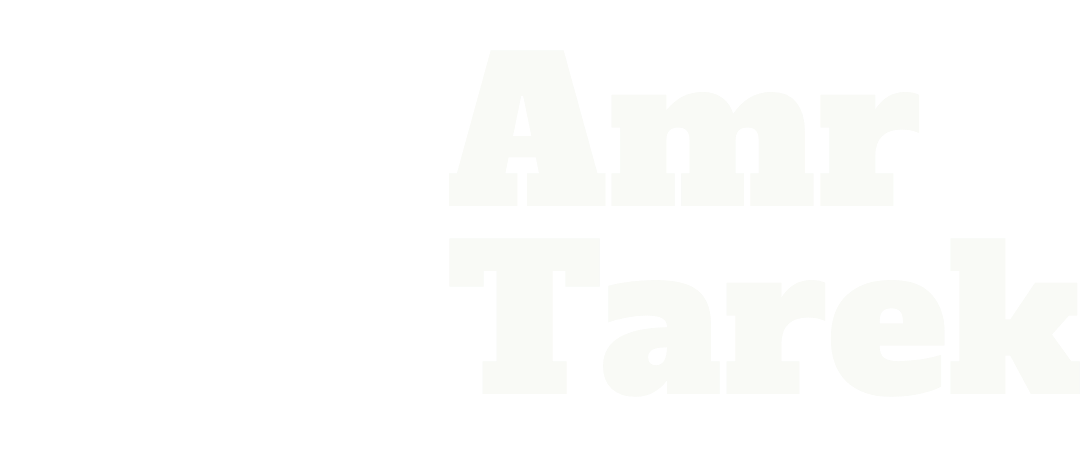
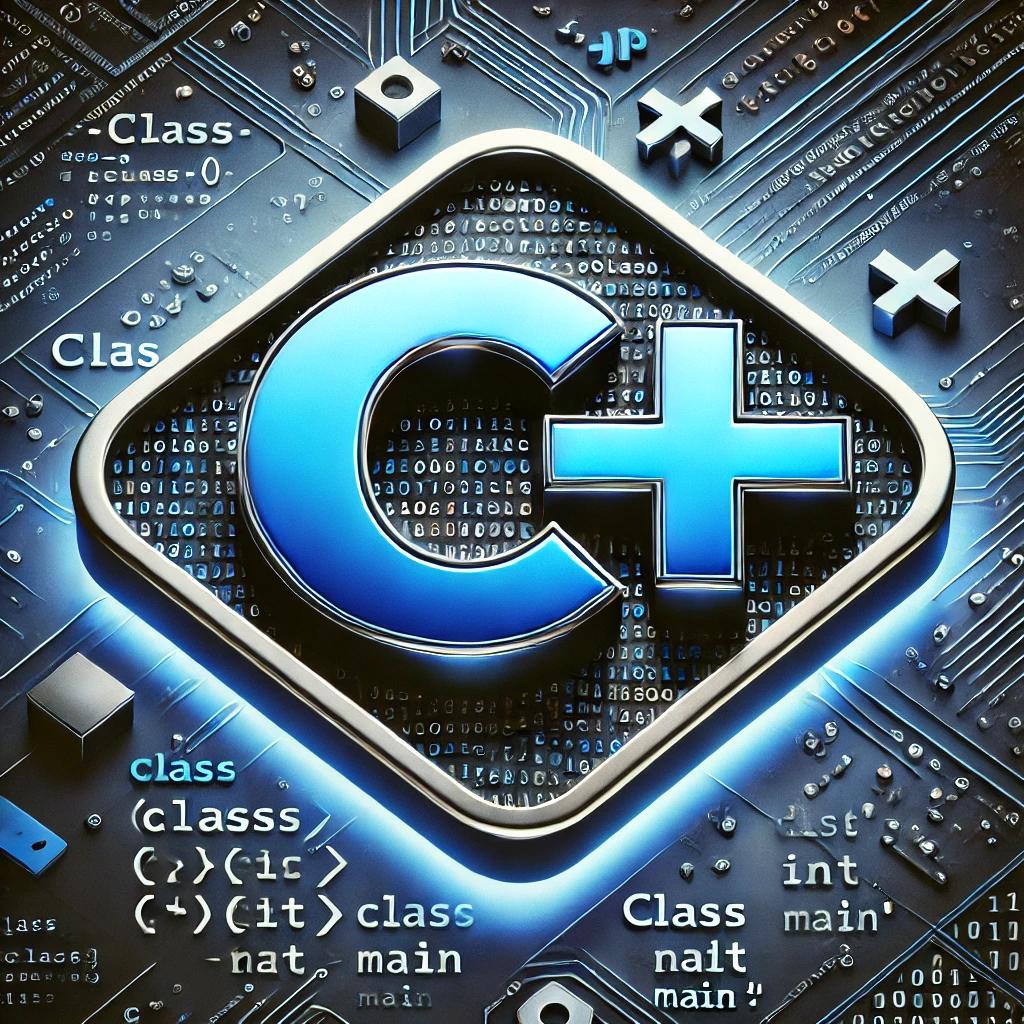
C++ STL - Algorithms
They are algorithms that already build to be used directly, rather than re-build them from scratch.
#include <algorithm>
std::vector<string> words;
// sort asending
std::sort(words.begin(), words.end());
// sort descending
std::sort(words.rbegin(), words.rend());
// reverse the container
std::reverse(words.begin(), words.end());
// it will count how many "Amr" string in the vector or any collection
int threes = count(begin(words), end(words), "Amr");
In C++, the <algorithm>
header provides a collection of functions designed for performing common algorithmic operations on sequences of data, such as sorting, searching, modifying, or manipulating elements in containers like arrays, vectors, and lists.
Some key functionalities included in <algorithm>
are:
1. Non-modifying sequence operations:
std::for_each
: Applies a function to a range of elements.std::find
: Searches for an element in a range.std::count
: Counts the number of elements in a range that match a value.std::mismatch
: Finds the first position where two ranges differ.std::equal
: Checks whether two ranges are equal.
2. Modifying sequence operations:
std::copy
: Copies elements from one range to another.std::swap
: Swaps the values of two elements.std::replace
: Replaces occurrences of a value in a range with another value.std::fill
: Fills a range with a specified value.std::remove
: Removes elements equal to a specified value from a range (logical removal).
3. Sorting and partitioning:
std::sort
: Sorts elements in a range.std::stable_sort
: Stable sort for elements.std::partial_sort
: Partially sorts a range, placing the smallest elements in order.std::partition
: Reorders elements in a range according to a predicate.
4. Searching:
std::binary_search
: Checks if a value exists in a sorted range.std::lower_bound
: Finds the first position where an element can be inserted without violating the order.std::upper_bound
: Finds the last position where an element can be inserted without violating the order.std::equal_range
: Returns the subrange where a value appears.
5. Heap operations:
std::make_heap
: Turns a range into a heap.std::push_heap
: Inserts an element into a heap.std::pop_heap
: Removes the largest element from a heap.std::sort_heap
: Sorts a heap.
6. Set operations (on sorted ranges):
std::set_union
: Computes the union of two sorted ranges.std::set_intersection
: Computes the intersection of two sorted ranges.std::set_difference
: Computes the difference between two sorted ranges.std::set_symmetric_difference
: Computes the symmetric difference of two sorted ranges.
7. Other algorithms:
std::min
,std::max
: Returns the smaller or larger of two values.std::clamp
: Constrains a value between two bounds.std::transform
: Applies a function to elements in a range and stores the result.