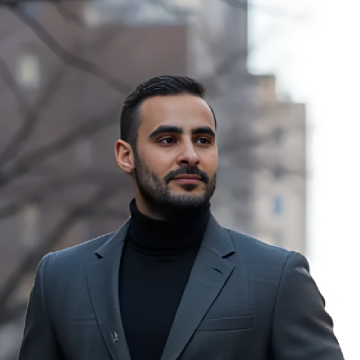
Amr Tarek
Software Engineer
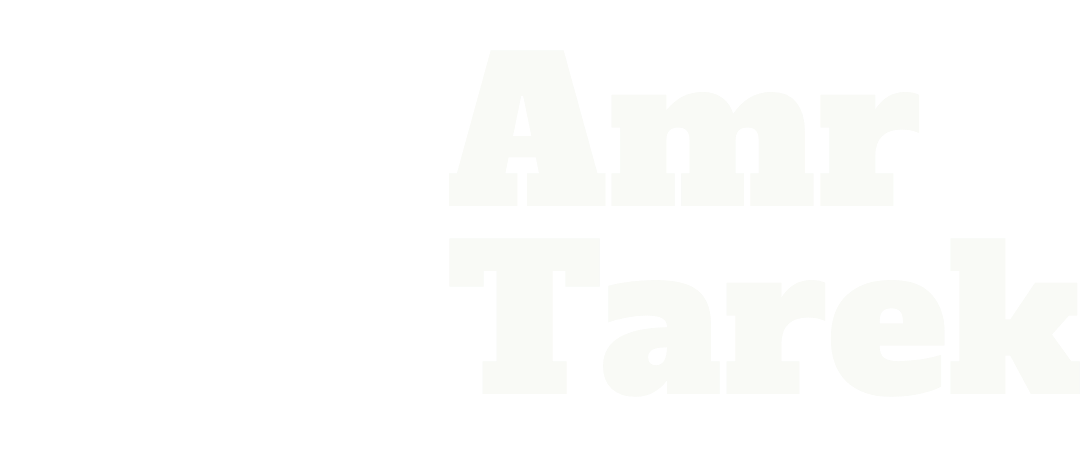
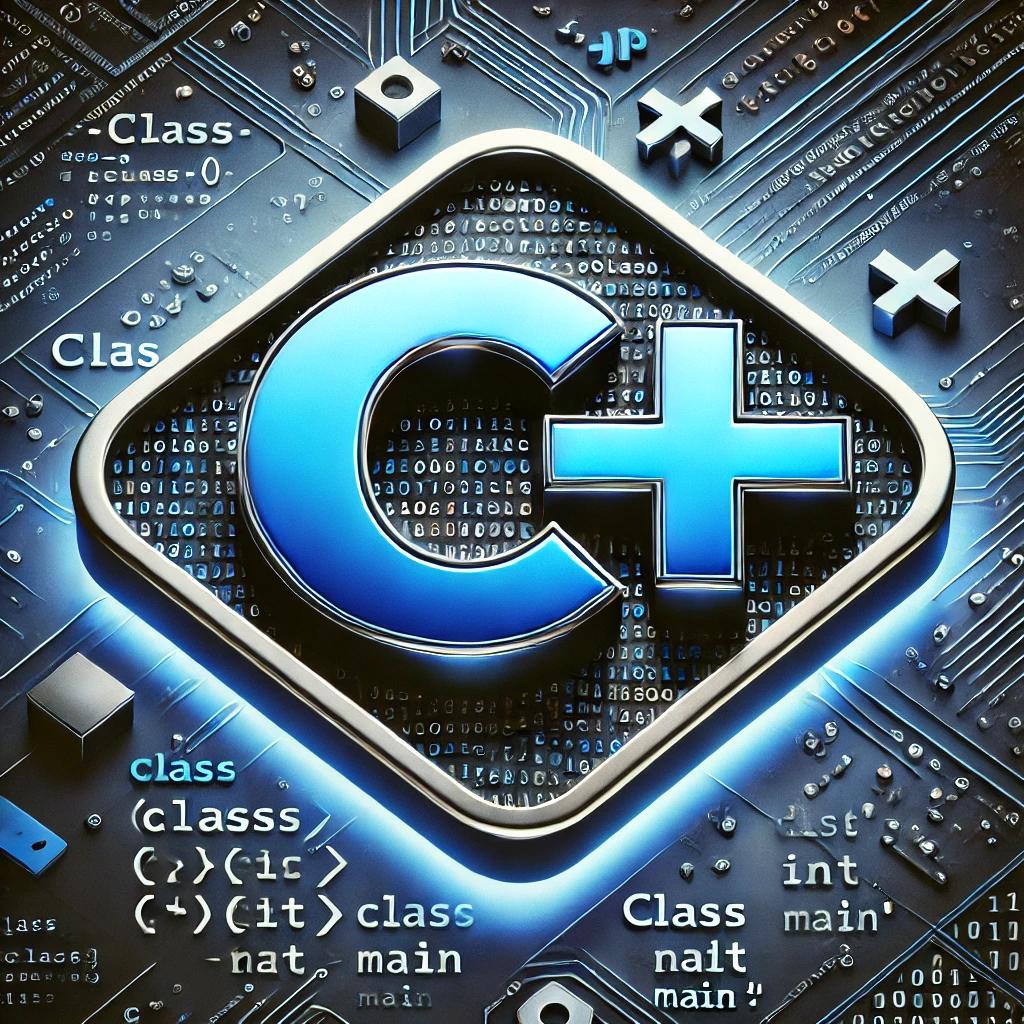
Standard C++ library
C++ Library is a library of code described by the C++ standard, and it delivers the standard implementation of C++, and it comes with two standard libraries: the old C library (Libc.lib), and the new C++ library (libcp.lib), so in simple words its a collection of classes and functions which are written in the core language, and it is updated every new standard for C++ every 3 years.
So, STD (Standard Library) does not refer to one library file but a collection, and it has been enhanced and incremented with every C++ version.
- The first C++ standard (C++98) the std has:
- STL (Standard Template Library) that containers and algorithms
- Strings library < string >
- I/O streams
- Then in (C++11) in 2011 the std extended to have:
- Regular expressions
- Lambda Expressions
- nullptr
- Smart pointers
- Move semantics and rvlaue reference
- constexpr
- Hash tables
- Random numbers
- Time Library
- Multithreading
- Then in (C++14) in 2014:
- Improved Smart pointers
- Improved Multithreading
- Binary Literals: 0b1010
- make_unique
- Adding Tuples, and type traits.
- Then in (C++17) in 2017:
- Adding String view
- Parallel Algorithm in the STL(Standard Template Library).
- Filesystem Library
- std::any, std::optional, and std::variant types.
Start with C++ STD
So the C++ standard library is a system library that already comes with your compiler package, and any call needs std namespace from the standard library and by default, the C++ linker links the std library.
c++ version (C++11, C++14, etc) specify which functions and classes is already in this std.
#include <iostram>
int main()
{
std::cout << "Hello\n";
std::cout << "Hello world" << "\n";
return 0;
}
it has in general two parts:
- The old library from C language called C STD
- The new library from C++ Language called C++ STL
The two parts are updating every C++ standard release.
C STD
The old library keeps being updated with the great number of functions and macros supporting a broad scope of functionalities that are related to the old C language and C++ does not duplicate the functionality of the standard C library, it uses the standard C library for many common functions like:
- the C String library
<cstring>
- the C Math library
<cmath>
You can note that it always starts with c, and you can find the full list in the cplusplus reference here C library. Each header from the C Standard Library is included in the C++ Standard Library under a different name, generated by removing the .h, and adding a 'c' at the start; for example, 'time.h' becomes 'ctime'.
#include <string.h> // the C header version.
#include <cstring> // the C++ version.
So for example to use printf
function which is defined in stdio.h
header in C language, the C++ version from it is in cstdio
header.
#include <cstdio>
int main()
{
printf("Hello world from C++");
return 0;
}
Strings
Strings was already added to C++ in C++ 98 and it is defined in the <string>
header. It provides a dynamic, flexible, and safer alternative to C-style strings (char[]
).
The std::string
class manages memory automatically, allows for a wide range of string manipulation functions, and avoids many of the pitfalls of C-style strings, like buffer overflows.
#include <string>
#include <iostream>
int main()
{
std::string str = "Hello"; // From a C-string
char ch = str[1]; // 'e'
char ch2 = str.at(1); // 'e', with bounds checking
std::string str2(str); // Copy constructor
std::string str3 = str1 + " World"; // Concatenation
std::cout << str.size() << std::endl; // Output: 5
std::cout << str.capacity() << std::endl; // Capacity (can be greater than 5)
std::string str = "Hello World";
str.append("!"); // "Hello World!"
std::string sub = str.substr(0, 5); // "Hello"
size_t pos = str.find("World"); // Returns index of "World"
str.replace(6, 5, "C++"); // "Hello C++!"
const char* cStr = cppStr.c_str(); // Convert to C-style string (const char*)
for (auto it = str.begin(); it != str.end(); ++it)
{
std::cout << *it; // Output each character
}
}
InputOutput Stream
The <iostream>
header was introduced as part of the C++ standard library in C++98 (the first ISO standard of C++).
<iostream>
is a C++ header that provides facilities for input and output (I/O) operations.
It defines objects, functions, and manipulators to handle streams for reading from and writing to standard input (cin
), standard output (cout
), and standard error (cerr
).
#include <iosstream>
int main()
{
int age;
std::cin >> age;
std::cout << "Hello, World!" << std::endl;
std::cerr << "Error occurred!" << std::endl;
std::clog << "Logging information..." << std::endl;
}
StringStream
std::stringstream
is a powerful tool in C++ for working with strings as if they were streams (like std::cin
and std::cout
).
It's particularly useful when you need to convert between strings and other data types or parse data from a string.
#include <sstream>
#include <iostream>
int main
{
// Convert string to number
std::string input = "123";
int number;
std::stringstream ss(input);
ss >> number; // converts string "123" to int 123
// Convert number to string
int num = 456;
ss << num; // Insert interger into the stream
std::string str = ss.str(); //convert the stream to string
// String parsing
std::string sentence = "Hello world C++";
std::stringstream ss(sentence);
std::string word;
// Output each word on a new line
while(ss >> word){
std::cout << word << std::endl;
}
// CSV parsing
std::string line = "John,25,180";
std::stringstream ss(line);
string name;
int age, height;
char comma;
ss >> name >> comma;
ss >> age >> comma;
ss >> height >> comma;
// String concatenate
int a = 10;
float b = 3.14;
std::string text = "Number:";
std::stringstream ss;
ss << text << " " << a << ", Pi: " << b;
std::string result = ss.str();
std::cout << result << std::endl;
// it will scape the new line for previous input.
std::cin.ignore();
// Will get the full line with spaces
std::getline(std::cin, result);
// using <iomanip> to set the precision for after the float point to 1 (0.0)
std::cout << std::fixed << std::setprecision(1) << result << endl;
}
std::stringstream
(from <sstream>
) builds on the concept of I/O streams provided by <iostream>
, but it operates on strings rather than console I/O. This allows treating strings like input/output streams.