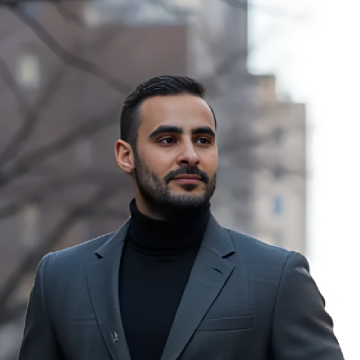
Amr Tarek
Software Engineer
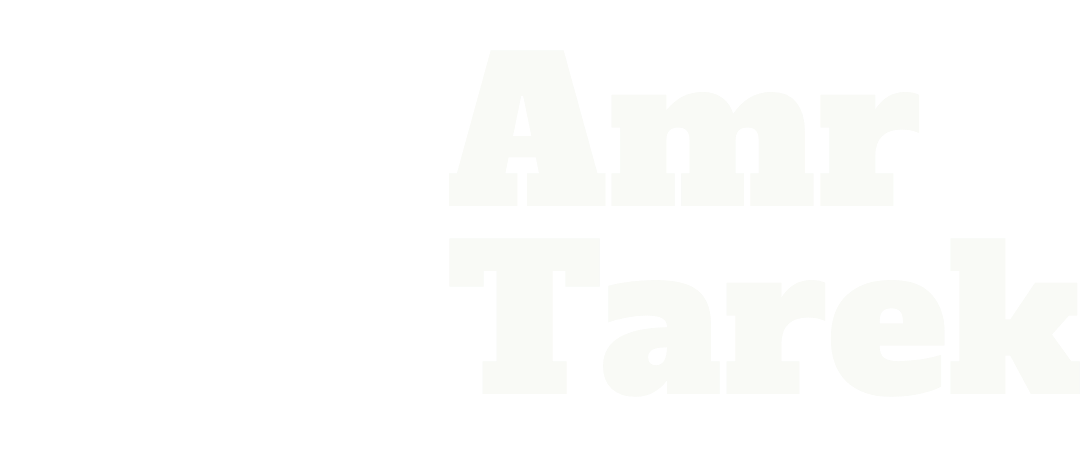
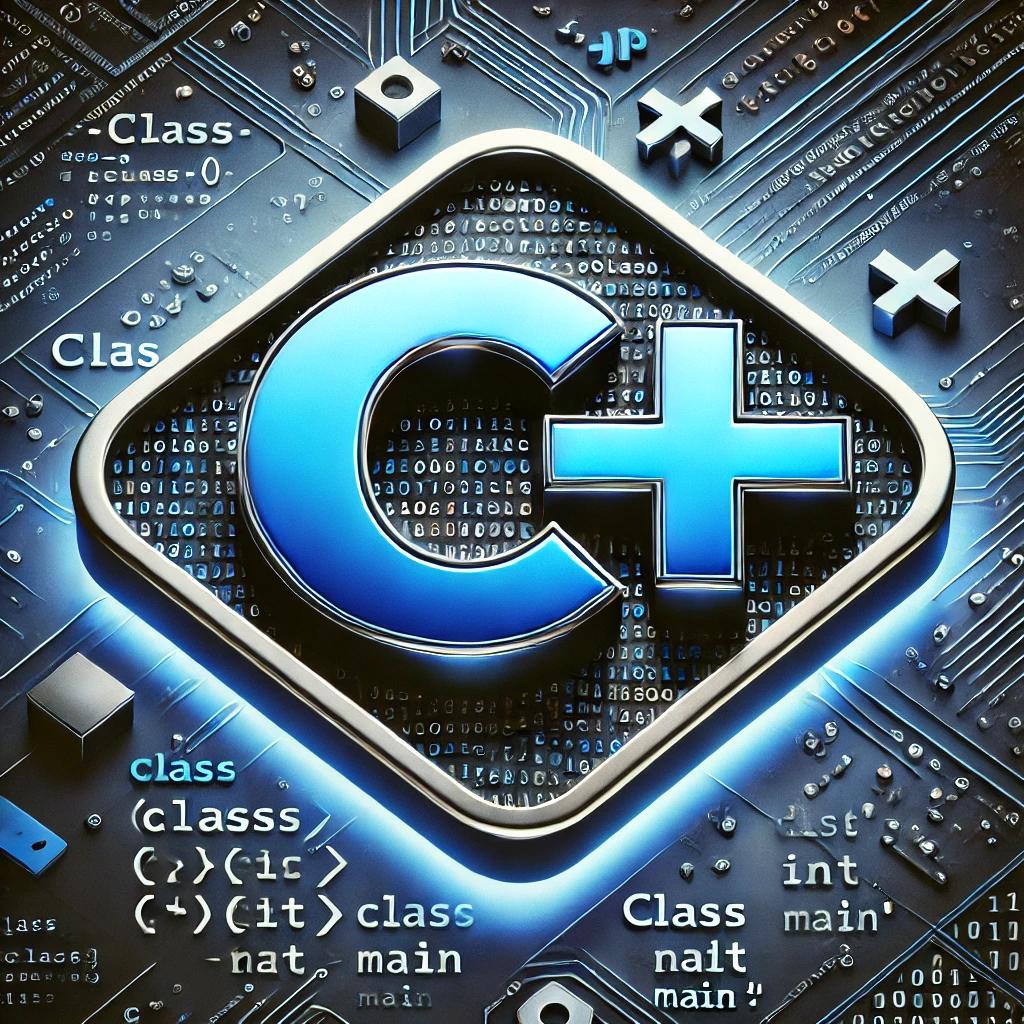
C++ Operator Overload
In C++, operator overloading allows you to redefine or "overload" most of the built-in operators to work with user-defined data types (like classes and structs).
To overload an operator, you define a function with a special name that includes the keyword operator
followed by the symbol for the operator you want to overload. This function can be a member function of a class or a non-member function.
Operators that can be overloaded
Almost all C++ operators can be overloaded, with a few exceptions. The operators that can be overloaded include:
- Arithmetic operators:
+
,-
,*
,/
,%
- Relational operators:
==
,!=
,<
,>
,<=
,>=
- Logical operators:
&&
,||
,!
- Bitwise operators:
&
,|
,^
,~
,<<
,>>
- Assignment operators:
=
,+=
,-=
,*=
,/=
,%=
,&=
,|=
,^=
,<<=
,>>=
- Unary operators:
++
,--
,-
,+
,*
,&
- Subscript operator:
[]
- Function call operator:
()
- Member access operators:
->
,.*
,->*
- Comma operator:
,
- Dereference operator:
*
- Address-of operator:
&
- New and delete operators:
new
,delete
,new[]
,delete[]
- Type cast operators:
typeid
,dynamic_cast
,const_cast
,reinterpret_cast
,static_cast
Operators that can not overloaded
There are a few operators in C++ that you cannot overload:
- Scope resolution operator:
::
- Member access operator:
.
- Member pointer access operator:
.*
- Conditional (ternary) operator:
?:
- Sizeof operator:
sizeof
- Typeid operator:
typeid
- Alignof operator:
alignof
- Noexcept operator:
noexcept
#include <iostream>
// Define a struct to represent a point in 2D space
struct Point {
int x, y;
};
// Overload the + operator for the Point struct
Point operator + (const Point& p1, const Point& p2) {
Point result;
result.x = p1.x + p2.x;
result.y = p1.y + p2.y;
return result;
}
// Function to display the coordinates of a point
void display(const Point& p) {
std::cout << "(" << p.x << ", " << p.y << ")" << std::endl;
}
int main() {
Point p1 = {3, 4}; // Initialize point p1
Point p2 = {1, 2}; // Initialize point p2
Point p3 = p1 + p2; // Use the overloaded + operator
std::cout << "p1 + p2 = ";
display(p3); // Output: (4, 6)
return 0;
}
Guidelines for Operator Overloading
- Maintain Intuition: The overloaded operator should behave in a way that is intuitive and natural to users of your class. Overloading
+
for a class should likely result in a meaningful addition, just as it does for built-in types.- Consistency: If you overload one operator, consider if it makes sense to overload related operators as well (e.g., if you overload
==
, consider also overloading!=
).- Return Values: For operators like
=
,+=
, or-=
, return*this
to allow chaining of operations.- Avoid Overloading Operators that Might Confuse Users: Some operators, like the comma operator
,
, might not always be suitable for overloading due to their subtle behavior.