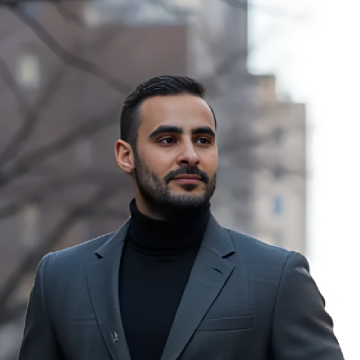
Amr Tarek
Software Engineer
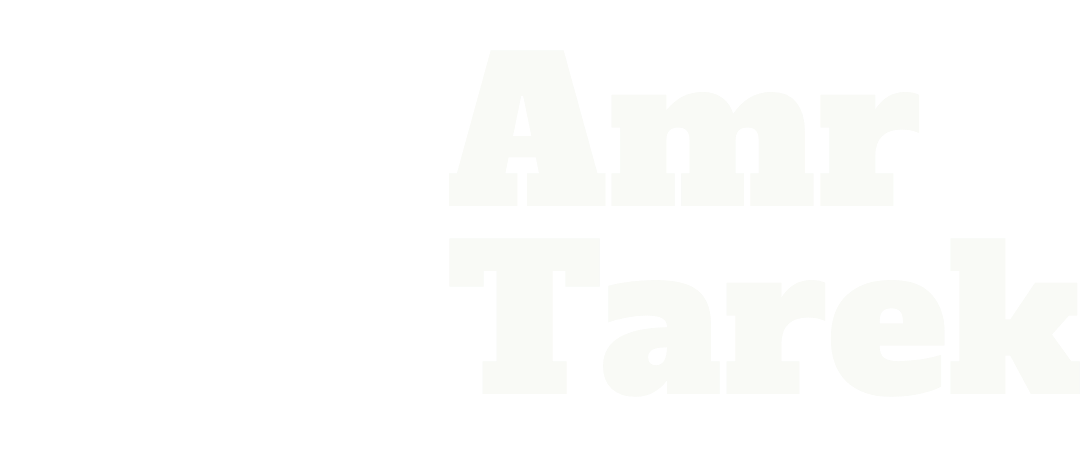
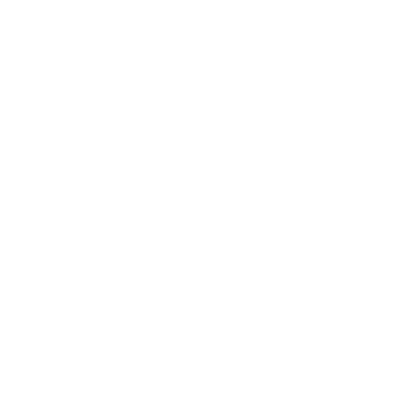
Amr Tarek
Docs
Programming Languages
Choosing the right programming language can significantly influence the success of a project. Among the myriad of languages available, C, C++, Python, and Rust stand out due to their unique features and widespread adoption.
Software Tools
A powerful set of essential software tools including Git, Docker, CMake, VS Code, Postman, and GitHub for modern development workflows.
Blog
Videos
About Me
I am Amr Tarek, an Embedded Software Engineer with over a decade of experience spanning Medical, Automotive, IoT, E-Banking, and Home Automation domains. I specialize in low-level firmware development, embedded Linux systems, device drivers, and cross-compilation toolchains. My goal is to create robust embedded solutions that enhance system reliability and user experience through efficient software architecture.
My professional journey includes leading-edge projects in signal processing using computer vision, real-time systems, home automation integration, and developing secure connectivity layers in automotive software. I am passionate about continuous learning and sharing knowledge through mentoring and technical content creation.
- Full NameAmr Tarek
- ResidenceNetherlands
- Websiteamrtarek.dev
- LinkedInlinkedin.com/in/amrtarek-dev
- LanguagesEnglish, Arabic
- AvailabilityFreelance / Contract
What I Do
Testimonials
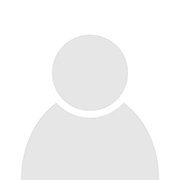
Amr is a top-tier embedded engineer. His contributions to our automotive software development improved system security and reliability significantly.
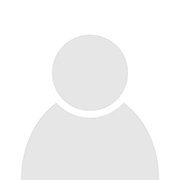
His knowledge in embedded Linux and driver development was vital for the success of our NatureConnect product line.